PROJECT: Deadline Manager
This portfolio serves to document my contributions to the CS2103T Software Engineering project - Deadline Manager. The project was developed by a team of 5 students in a span of 6 weeks.
1. Overview
Deadline Manager is a tool written for students in NUS School of Computing to better manage their deadlines and priorities. Interacting through a Command Line Interface (CLI), users can easily manage their schedules and focus on what is most important to them.
Written in Java, Deadline Manager builds upon AddressBook - Level 4, a code base originally designed to teach Software Engineering principles.
For more information and to do a test run, please visit our home page [on GitHub].
2. Summary of Contributions
My key contributions to the project includes:
-
Major enhancement: Added the ability to sort tasks of the Deadline Manager in a user-defined manner.
-
What it does: It allows the users to order the tasks using a user-defined sort expression. A single sort expression can be composed of multiple comparators, where each comparator is based on a certain attribute(Example - Name, Due Date, Priority) of the task.
-
Justification: This feature is critical to the Deadline Manager because if a user has lots of deadlines/tasks due and they only want to focus on the most important ones. The sort functionality enables them to view those deadlines which are more important at the top of the list followed by the those which are less important.
-
Highlights: The sort functionality supports chaining of comparators, which enables the user to fine-tune how they want to order the tasks. Example - If a user has a lot of tasks with the very close due dates, the sort function allows them to first sort by due date and then by priority. This sorts all the tasks by due date first and all those tasks with the same due date are sorted by priority within themselves.
-
Credits: Referenced Java Comparator Chaining and Regex fine-tuning
-
-
Minor enhancement: Implemented natural comparison for some of the attributes of the task (Ex. Priority, Name)
-
What it does: Allows comparison for the different attributes of the task. Primarily used for filtering and sorting of the tasks.
-
-
Code contributed: CS2103T Project Code Dashboard
-
Other contributions:
-
Project management:
-
Contributed to user stories on GitHub issue tracker (User Stories)
-
Manage milestones on GitHub
-
-
Significant modification of Address Book’s code base for use in Deadline Manager:
-
Renaming of classes and variable names from Address Book to Deadline Manager: #117
-
-
Community:
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
3.1. Sorting a list of tasks : sort
Sorts the lists of all the tasks which the user is currently viewing. Generally meant to be used in combination with filter
.
When this command is used, Deadline Manager will sort all the currently displayed tasks according to the user-specified comparison method.
Sort comparators are the core of the sort
command. Each sort comparator specifies a particular attribute (Example - name
, priority
) and a comparison direction, i.e ascending or descending.
The entire sorting command is composed of a chain of sort comparators. This chain helps the user to define which tasks to show earlier and which ones to show later.
The sort command results in a stable sort, i.e if two deadlines are equal according to the sort comparators then their relative order before the sort command and after the sort command remains the same. |
Format: sort SORT_COMPARATOR [SORT_COMPARATOR]…
Examples:
-
sort n>
Sorts the current list of tasks in view in descending order by name, where sorting is done in alphabetical order.
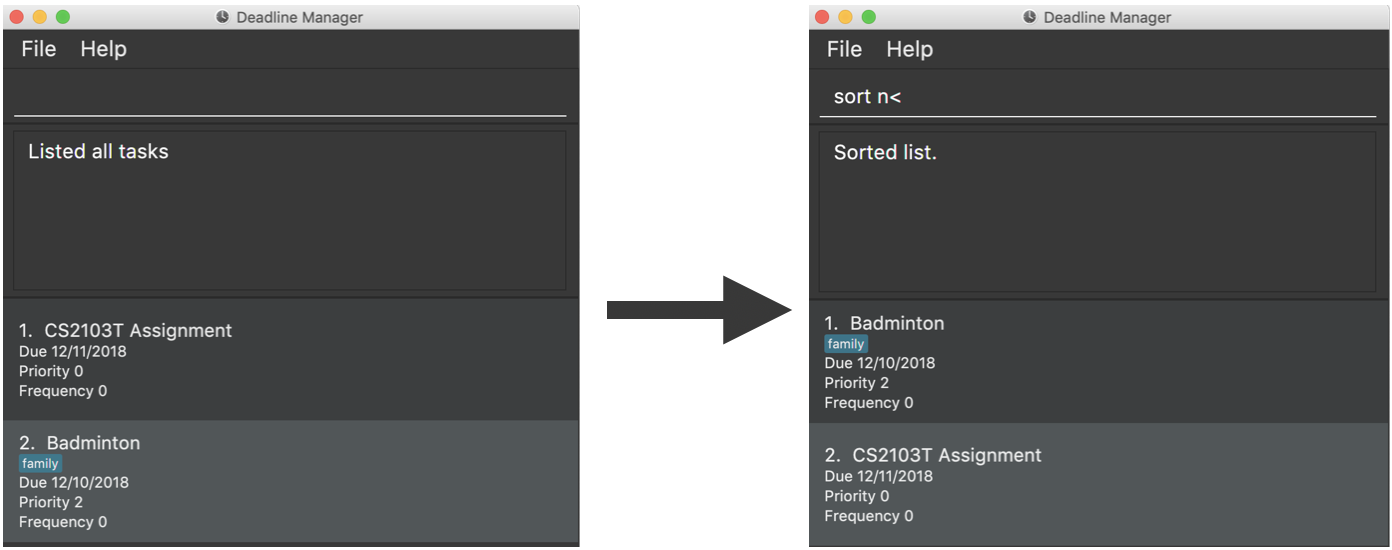
-
sort due< name>
Sorts the current list of tasks in view in ascending order by due date, where ties are broken by descending order of names. -
sort priority<
Sorts the current list of tasks in view in ascending order by priority. -
sort frequency<
Sorts the current list of tasks in view in ascending order by frequency. -
sort tag<{ cs2100 family cs2103t }
Sorts the current list of tasks in view in ascending order by tags. In this case all the tasks with the tagcs2100
will be placed before those tasks with the tagfamily
, finally followed by those tasks with the tagcs2103t
. -
sort n< tag<{
This is invalid syntax since the curly braces have not been closed.

The above figure shows the error message when user feeds in an invalid command.
In case a task does not belong to any of the tags mentioned in the sort comparator it will be placed at the bottom of the sorted list. |
In case a task contains more than one tag specified in the sort comparator, then it will be sorted according to those tags first which place it formerly in the sorted list. |
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
4.1. Sort feature
The sort command enables the user to sort the tasks currently being shown according to a user-defined custom comparator.
It is made up of two main components - SortCommandParser
and SortCommand
4.1.1. Current implementation
The sort command is facilitated by VersionedTaskCollection
.
The sort command exposes the operation updateSortedTaskList
to sort the task list.
It is the responsibility of SortCommandParser
to parse the user input into a comparator which can compare between two tasks.
Then the comparator is passed onto the SortCommand
which sorts the versionedTaskCollection
according to the comparator.
A NullPointerException is raised in case the SortCommand receives a null comparator.
|
4.1.2. Sequence flow
Given below is a sequence of steps, illustrating the interaction between SortCommandParser
, SortCommand
and ModelManager
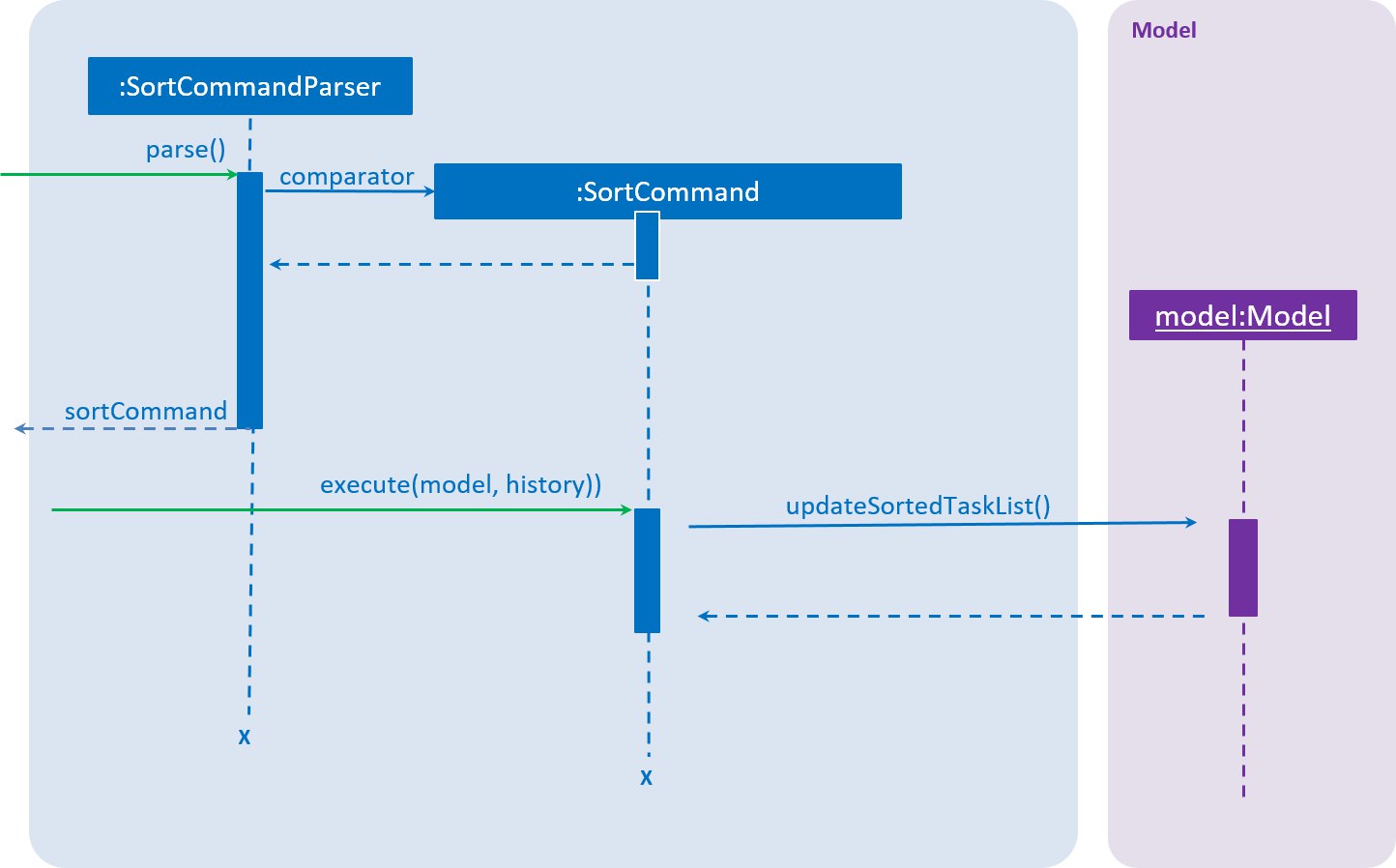
-
The user executes the sort command along with providing a user-specified comparator.
-
The
SortCommandParser
receives the command with the user comparator given as a string. -
The
SortCommandParser
parses the string into a valid comparator and calls theSortCommand
with this comparator provided. -
The
SortCommand
calls theupdateSortedTaskList
API method ofModelManager
-
The method
updateSortedTaskList
sorts theversionTaskCollection
using JavaFx sort method
4.1.3. Implementation of sort parser
SortCommandParser
implements Parser<>
interface. The most essential portion of SortCommandParser
, which is chaining of comparators is shown below:
public SortCommand parse(String args) throws SimpleParseException {
Comparator<Task> comparator = Comparator.comparing(Task::getClass, (a, b) -> {
return 0; // a default comparator which compares every task equal
});
// argumentsArray is an array of strings referring to all the arguments given to the sort command.
for (String arg: argumentsArray) {
final String taskField = arg.substring(0, arg.length() - 1);
final char comparisonChar = arg.charAt(arg.length() - 1);
switch(taskField) {
case "n": // fallthrough
case "name": {
if (comparisonChar == '<') {
comparator = comparator.thenComparing(Task::getName);
} else {
comparator = comparator.thenComparing(Taks::getName, Comparator.reverseOrder());
}
break;
}
case "p": // fallthrough
case "priority": {
if (comparisonChar == '<') {
comparator = comparator.thenComparaing(Task::getPriority);
} else {
comparator = comparator.thenComparing(Task::getPriority, Comparator.reverseOrder());
}
}
// ... consists of case for other task fields as well
}
}
return new SortCommand(comparator);
}
4.1.4. Design considerations
4.1.4.1. Aspect: Which task list to sort
-
Alternative 1 (current choice): The entire
versionedTaskList
is sorted using JavaFx inbuilt sort method.-
Pros: Easy to implement.
-
Cons: Slow in performance.
-
-
Alternative 2: The sorting is done ONLY on the viewable
filteredTasks
list.-
Pros: Fast in terms of performance.
-
Cons: Requires significant changes to the codebase since sorting the
filteredTasks
requires it to be modifiable.
-
4.1.4.2. Aspect: How to sort for tags
-
Alternative 1 (current choice): The sort command takes in user input so that the user can specify the priority order of tags.
-
Pros: Provides immense flexibility to the user. Also follows Law of Demeter / Law of Least Knowledge since the
Tag
model itself does not know that it can be compared. -
Cons: The flexibility comes at the cost of making sorting by tags complex for newbie users.
-
-
Alternative 2: The sorting is done in a pre-defined manner, example sorting tasks according to their alphabetical order of tags.
-
Pros: Easier to implement and simpler for newbie users.
-
Cons: Not useful for the user in many situations. Disobeys the Law of Demeter / Law of Least Knowledge since the
Tag
model will know that it can be compared with other tags.
-